Reference Works
This is a list of my projects and codes I have worked on. I have collected here all of them, which I think are relevant or worthy to show. This means that I have excluded the projects, which are not .Net based or I have developed them in my earlier career and I feel like they would not showcase my current skills. Earlier I was highly involved into sports betting, for which I created a few small applications. These are also not included here.
The list contains my hobby and work projects and features. The number of hobby projects are decreasing constantly, since I try to integrate them to this website. In every case, I tried to include a short description about the goal of the project and about the general architecture of the code. I have also attached the source code, where I could.
This ASP.Net Core MVC website
This website is a pure ASP.Net Core MVC project. It is hosted in Azure, where it has a database connected to it. The custom domain and security certificates are provided by Cloudflare. Here is an image about the architecture:
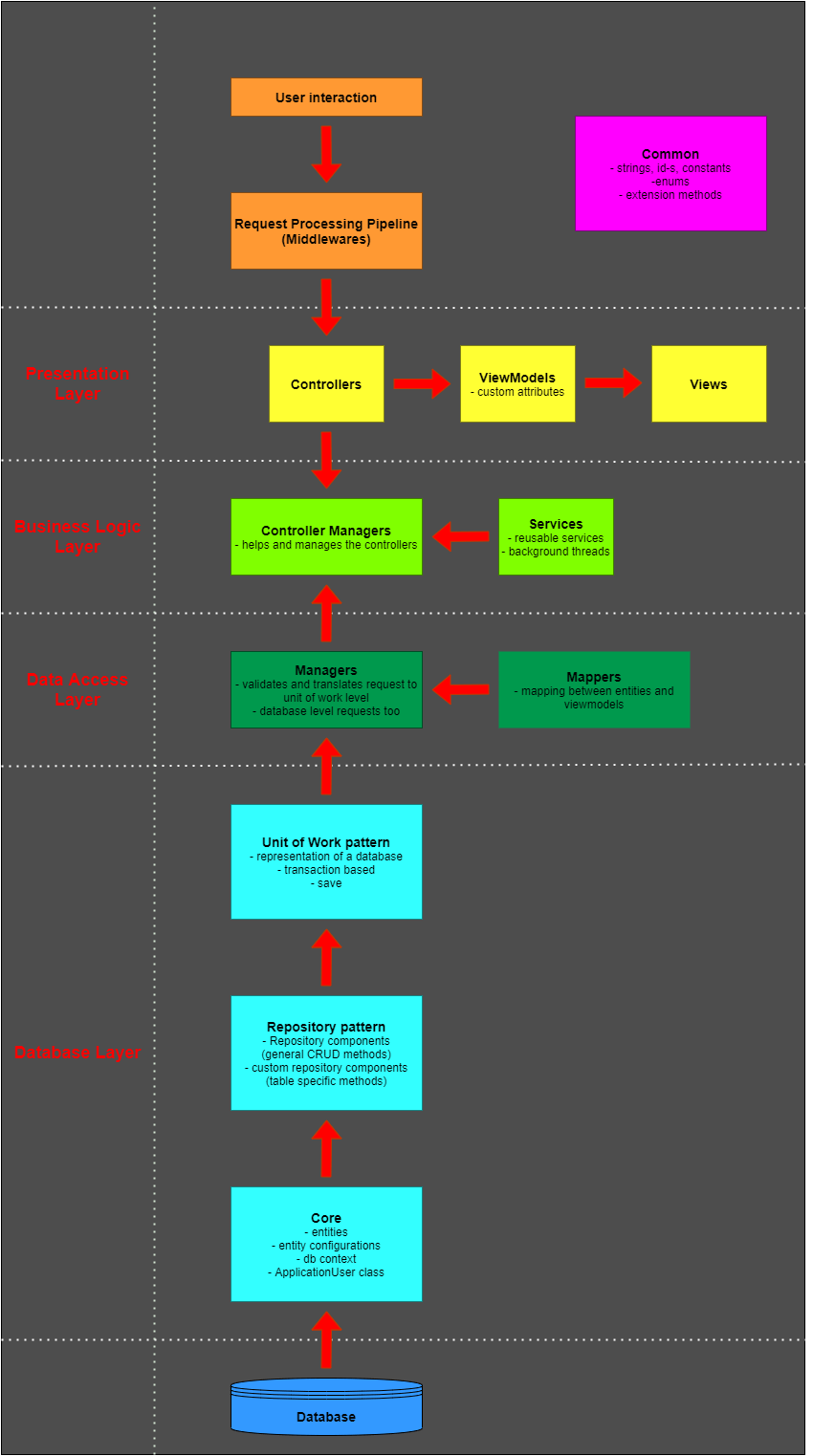
The website has two hosted background services. Both are connected to the task module, where the user can create reoccurring items, and see the daily tasks for a given date. One of the worker services, it is populating the database in the morning with the tasks for that day. The other one is sending email notifications about the daily tasks to the users, who are enabling this feature in the settings.
The website main parts are the controllers, views and the viewmodels. The default routing is used to direct the requests to the controllers, which are displaying the views and communicating the data over the viewmodels. The view files are cshtml files, so they consists mostly of html, css and javascript. Each controller has a controller manager class, which is responsible for processing the data for the controllers. This means interacting with the database layers and also using additional services.
The database layer is connected to the business logic layer with a data access layer, which provides abstraction between the two. This means using the unit of work pattern and mapping database entities to viewmodels and models used in the frontend. The database layer also uses the repository pattern, so a full wrapping happens above the entity framework core. I am using the code first workflow in the database layer.
There are parts of the website, which I don't want to enable for everybody or it is related to only one person. That is why there is a registration and login process implemented. As external login, one can use Facebook and Google too. The accounting service I am using is the built in identity service.
I have added logging for both app insights and files. The sensitive secrets and keys are in Azure key vault. In Azure, I am in a lower app service plan, which results that the "Always on" settings cannot be enabled. This settings should keep the website alive, when it is inactive for a certain time. This causes two problems for me. On one hand, at the first loading, it has to restart the website and rebuild the code, so the first loading time is around one entire minute. On the other hand, the background services also cannot do their jobs, since the app is usually inactive in the early hours of the days. To overcome these problems, I am using uptimerobot.com to ping the website in every half an hour and does not let it to go idle.
There is also a unit testing project, which is not shown on the architecture map. I try to create unit tests as many as I feel it is necessary. The unit tests help me to find smaller errors or missed cases in the program code, and also encourage to make more separated, loosely coupled code.
Source code: githubGeocoder Windows service with sql service broker
This is a project from work, where I had to create a Windows service, which can transform addresses from multiple databases and tables to geocoordinates.
This was the problem we had at that time. There was an sql server with lots of different customer databases. Each database had a table with the locations. These locations were addresses, so they had properties like city, postal code, street and house number. For other services, we needed to transform these addresses to longitude and latitude values and place these coordinates back to the locations table. The data of these addresses came from various sources, so there was not really a good way to geocode the addresses when they got into the database. That was the reason of using the sql service broker feature. This feature enables a queue mechanism inside the sql server. Therefore, with using triggers on the location tables, I could create a queue, where the message contained the data to geocode and the source of the data, where the geocoordinates must be written back. The Windows service eventually read the messages from service broker queue, calculated the geocoordinates with Google and wrote back the values into the locations table of the proper database. All this happened inside one transaction, securing to not lose any data.
The project was made in .Net core. It used native sql connection classes, transactions and stored procedures to interact with the database. These required lots of hard coded strings, like the sql scripts, connection strings and others. So the project used the built in dependency injection container with the options pattern. The geocoding Google service was accessible by an API and that called the Google services directly. The API was already there, I just had to call and process the results. We also needed statistics and monitoring about the geocoding, and this was done with Azure and the TelemetryClient class. Since the geocoding could have had huge load, it must have been made to a multi thread service, so eventually it was able to process around 1000-3000 addresses in every minutes depending on the settings. This required multi threading, especially in the statistics tracking.
Even though the project was a smaller one, it used a lot of interesting techniques. The sql service broker feature was completely new to me as well. This service is still in use.
UWP project for push notifications
This was a proof of concept project in work, but was never finished as product and used in production. The goal was simple, send push notifications to mobile and desktop devices.
The project had a server side, which was a web API basically. This had a controller with actions like register device, delete device, get registered devices and send push notifications. The project used the Azure Notifications Hub, but the messages were externally stored in a Cosmos db as well. A requirement was that the user could setup when to receive notifications, so we stored the messages and sent them in the proper time. A task was in charge to do this in the background. The access of the controller was authenticated by the company's identity service. So the clients to access the controller must include a proper token.
The client part of the project was not implemented in all platforms. To make life easier, I did it only for windows desktop. This was a very simple UWP application. In general, everything was handled in the API, so the client could only call the endpoints and not worry about anything. There is only one thing, which must be done by the client. This is the channel uri, which represents the way the Azure Notifications Hub can identify and communicate with the client device. To receive and process the push notifications, the app must subscribe to the events of this channel. For all supported platforms, there is a documentation how to do it.
I have added a test application, which was extracted from the web API we would have used. It is a simple console application and I made a lot simpler than it was for demonstration purposes. However, it will not work actually, because the connection string and the channel uri to the client is missing.
Source code: NotificationTest.rarStrategy based card collection game in Unity with C# server
This project is a huge one and it is far from finished state. In fact, it barely has any functionality currently. This is one of my first own ideas and I started this a couple of years ago, but never went to deep inside the development of it. Most likely in the future I will continue and I want to create a prototype. Probably just for fun, for myself or maybe this will somehow be part of my thesis in the university.
The game would be a mix of Hearthstone and the old Heroes of Might and Magic. So the players can collect battle units and war leaders and fight with another player on a hexagon based map. The theme is the medieval era, because I like history in general and know a lot about those times.
The game server is written in C#. It uses Autofac as the DI container. The database has repository and unit of work patterns and follows the code first workflow. As for logging, it uses Serilog. The communication happens via TCP protocol and messages are serialized in xml format.
The client side is written in Unity engine with C#.
I attached a code sample from the game. Most of it was written years ago and to continue it, probably it needs lots of refactoring.
Source code: Game.rarSending sms with Twilio
This was just a small proxy class over the Twilio .Net sdk. The code was pretty much based on the Twilio documentation. Basically it contained an initialization, a send method, logging and proper exception handling. Nothing too fancy. I am planning to use this service in my own website somewhen in the future.
OpenID Connect authentication in javascript
This was not a separate project, but rather a small feature implemented in work. It wasn't even strictly .Net related, since this was developed inside a cordova mobile application. Cordova is javascript based and the plugin I used, it is that also. The reason why I mention it here is, because the oidc protocol is popular and can be used in any programming language. Also to use it, I had to understand the whole workflow of it.
The identity server, which implemented this type of protocol, it already existed, so the javascript implementation was very simple in theory, since I just had to configure the oidc plugin to use the id server. The application had another type of authentication mechanism, so this had to be implemented in sync with the existing method. The user could initiate the oidc login by clicking a button, which showed a website inside the application, where the user could enter the credentials and log in.
Intercom messenger in WPF and mobile projects
Intercom is a customer business messenger with other functionalities as well, like app tour, tutorials, help articles. In work, we wanted to enhance the customer help center experience with this service. Therefore, it had to be integrated into different technologies, apple, android, javascript and C# WPF. Intercom has plugins to use in mobile environments, which is very nice and easy to use. However, it is not intended for desktop applications. Intercom has an API, where the basic things can be done, like customer registration and accessing the conversations. So to implement it in desktop, I used this API and created a browser window, to which I could supply javascript to mimic a browser like behavior. With this technique, I could implement intercom in all platforms with messenger, help articles and showing the unread messages automatically.